Version: 2.1
By: Berka
Introduction
Now, your crazy project is able to download and upload files on ftp or http servers.
Features
- No external files/libs, only Apis
- Fast transfers
- ProgressBars...
- Ftp and http support
Screenshots
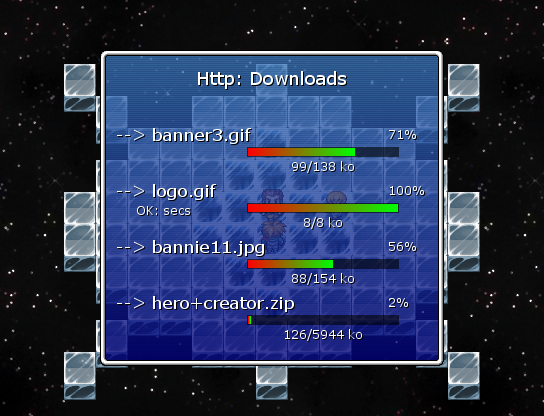
Demo
RMVX
RMXP
Script
The Net module:
Scene Example:
Code:
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
#Â Â Â Â Â Â Â Â Â Â Download & Upload Files with RGSS
#  par berka           v 2.1          rgss 2
#Â Â Â Â Â Â Â Â Â Â Â Â Â [url=http://www.rpgmakervx-fr.com ]http://www.rpgmakervx-fr.com [/url] Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# thanks to: [url=http://www.66rpg.com]http://www.66rpg.com[/url] for documentation on wininet
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# ! do not use ftp which contains privates data
# ! this scripts need ftp account information !
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# Ftp :
#  â–¼ receive :
#Â Â Â Â Â Â Net::Ftp.download("dir_on_ftp/file.zip","./Graphics")
#  â–¼ send :
#      Net::Ftp.upload("./Graphics/file.zip","§dir_on_ftp/file.zip")
#  â–¼ make directory :
#Â Â Â Â Â Â Net::Ftp.mkdir("/dir_on_ftp")
# Http :
#  â–¼ receive :
#Â Â Â Â Â Â Net::Http.download("url","./Graphics")
#  â–¼ total octets downloaded :
#Â Â Â Â Â Â Net::HTTP.dloaded
#  â–¼ size of file :
#Â Â Â Â Â Â Net::HTTP.size("test.zip")
#  â–¼ % dl progress :
#Â Â Â Â Â Â Net::HTTP.progress("test.zip")
#  â–¼ transfer time:
#Â Â Â Â Â Â Net::HTTP.temps("test.zip")
#  â–¼ list files :
#Â Â Â Â Â Â Net::HTTP.transfers
#  â–¼ file loaded? :
#Â Â Â Â Â Â Net::HTTP.loaded?("test.zip")
#  â–¼ octets transfered :
#Â Â Â Â Â Â Net::HTTP.transfered
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
module Berka
 module NetError
  ErrConIn="Unable to connect to Internet"
  ErrConFtp="Unable to connect to Ftp"
  ErrConHttp="Unable to connect to the Server"
  ErrNoFFtpIn="The file to be download doesn't exist"
  ErrNoFFtpEx="The file to be upload doesn't exist"
  ErrTranHttp="Http Download is failed"
  ErrDownFtp="Ftp Download is failed"
  ErrUpFtp="Ftp Upload is failed"
  ErrNoFile="No file to be download"
  ErrMkdir="Unable to create a new directory"
 end
end
include Berka::NetError
module Net
 W='wininet'
 SPC=Win32API.new('kernel32','SetPriorityClass','pi','i').call(-1,128)
 IOA=Win32API.new(W,'InternetOpenA','plppl','l').call('',0,'','',0)
 IC=Win32API.new(W,'InternetConnectA','lplpplll','l')
 print(ErrConIn)if IOA==0
 module FTP
  FSCD=Win32API.new(W,'FtpSetCurrentDirectoryA','lp','l')
  FGF=Win32API.new(W,'FtpGetFileA','lppllll','l')
  FPF=Win32API.new(W,'FtpPutFile','lppll','l')
  FCD=Win32API.new(W,'FtpCreateDirectoryA','lp','l')
  module_functionÂ
  def init
   #-=-=-=-=-=-=-=-=-=-=-=-=-=-=-#
   ftp="ftp.server.com"    #
   port=21            #  Modify !
   identifiant="user"      #
   motdepasse="password"    #
   #-=-=-=-=-=-=-=-=-=-=-=-=-=-=-#
   @fb=IC.call(IOA,ftp,port,identifiant,motdepasse,1,0,0)
   ftp,port,identifiant,motdepasse=[nil]*4 # clear ftp ids !
   (print(ErrConFtp))if @fb==0
  end
  def download(ext,int='./')
   init if @fb.nil?Â
   c=ext.split('/').pop
   if FSCD.call(@fb,ext.gsub(c,''))!=0
    print(ErrDownFtp)if FGF.call(@fb,c,"#{int}/#{c}",0,32,2,0)==0
   else
    print(ErrNoFFtpIn)
   end
  end
  def mkdir(rep)
   init if @fb.nil?
   print(ErrMkdir)if FCD.call(@fb,rep)==0
  end
  def upload(int,ext)
   init if @fb.nil?
   if FSCD.call(@fb,ext)&&File.exist?(int)
    print(ErrUpFtp)if FPF.call(@fb,int,ext,2,0)==0
   else
    print(ErrNoFFtpEx)
   end
  end
 end
 #-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
 module HTTP
  IOU=Win32API.new(W,'InternetOpenUrl','lppllp','l')
  IRF=Win32API.new(W,'InternetReadFile','lpip','l')
  ICH=Win32API.new(W,'InternetCloseHandle','l','l')
  HQI=Win32API.new(W,'HttpQueryInfo','llppp','i')
  module_function
  def sizeloaded(i=0);@read[i];end
  def transfered;@dloaded;end
  def transfers;@dls;end
  def progress(i=0);(@read[i].to_f/@size[i]*100);end
  def loaded?(i=0);@read[i]>=@size[i];end
  def temps(i=0);@tps[i];end
  def size(i=0);@size[i];end
  def download(url,int='./')
   @dloaded||=0;@dls||={};@i||=-1;@size||={};@read||={};@tps={}
   a=url.split('/');serv,root,fich=a[2],a[3..a.size].join('/'),a[-1]
   print(ErrNoFile)if fich.nil?
   @dls[fich]=Thread.start(url,int){|url,int|txt='';t=Time.now
   ErrConHttp if(e=IC.call(IOA,serv,80,'','',3,1,0))==0 Â
   f=IOU.call(IOA,url,nil,0,0,0)
   HQI.call(f,5,k="\0"*1024,[k.size-1].pack('l'),nil)
   @read[fich],@size[fich]=0,k.delete!("\0").to_i
   loop do
    buf,n=' '*1024,0
    r=IRF.call(f,buf,1024,o=[n].pack('i!'))
    n=o.unpack('i!')[0]
    break if r&&n==0
    @read[fich]=(txt<<buf[0,n]).size
   end
   (File.open(int+fich,'wb')<<txt).close
   @dloaded+=@read[fich]
   ICH.call(f)
   @tps[fich]=Time.now-t
   sleep(0.001)}
  end
 end
end
Â
Code:
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# Â Â Â Â Â Â Â Â Â Â Download & Upload Files with RGSS
#  par berka            v 2.1           rgss 2
#              www.rpgmakervx-fr.com                     Â
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# Â Â Â Â Â Â EXAMPLES !
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
class Window_Barre<Window_Base
 Col1=Color.new(255,0,0)
 Col2=Color.new(0,255,0)
 def initialize
  super(100,50,344,316)
  @dls=Net::HTTP.transfers
  refresh
 end
 def refresh
  i=0
  self.contents.clear
  self.contents.font.size=22
  self.contents.draw_text(-16,0,self.width,WLH,"Http: Downloads",1)
  self.contents.font.size=20
  @dls.each_key{|file|
  self.contents.draw_text(0,56*(i+=1),200,WLH,"--> #{file}")
  self.contents.fill_rect(131,56*i+25,152,10,Color.new(0,0,0,150))
  self.contents.font.size=14
  rat="#{Net::HTTP.sizeloaded(file)/1024}/#{Net::HTTP.size(file)/1024} ko"
  self.contents.draw_text(132,56*i+32,150,WLH,rat,1)
  s=Net::HTTP.temps(file)
  self.contents.draw_text(20,56*i+20,self.width,WLH,"OK:#{s} secs")if Net::HTTP.loaded?(file)
  pr=Net::HTTP.progress(file)/100
  self.contents.draw_text(272,56*i,self.width,WLH,"#{(pr*100).to_i}%")rescue nil
  self.contents.gradient_fill_rect(132,56*i+26,pr*150,8,Col1,Col2)
  self.contents.font.size=20}
 end
end
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
class Download<Scene_Base
 def start
  super
  @fond=Spriteset_Map.new
  Net::HTTP.download('http://download59.mediafire.com/313lzxpdytpg/gyok0vgmm2m/hero+creator.zip')
  Net::HTTP.download('http://www.google.fr/intl/fr_fr/images/logo.gif')
  Net::HTTP.download('http://www.rpgrevolution.com/forums/banner3.gif')
  Net::HTTP.download('http://i42.servimg.com/u/f42/12/32/03/86/bannie11.jpg')
  @barre=Window_Barre.new
 end
 def terminate
  super
  @barre.dispose
  @fond.dispose
 end
 def update
  super
  @barre.refresh
 end
end
Â
def telecharger
 Net::HTTP.download('http://download59.mediafire.com/313lzxpdytpg/gyok0vgmm2m/hero+creator.zip')
 Net::HTTP.download('http://www.google.fr/intl/fr_fr/images/logo.gif')
 Net::HTTP.download('http://www.rpgrevolution.com/forums/banner3.gif')
 Net::HTTP.download('http://i42.servimg.com/u/f42/12/32/03/86/bannie11.jpg')
end
Â
The Net module
The Scene Example:
Code:
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# Â Â Â Â Â Â Â Â Â Download & Upload Files with RGSS
#  par berka            v 2.1          rgss 1
# Â Â Â Â Â Â Â Â Â Â Â Â [url=http://www.rpgmakervx-fr.com ]http://www.rpgmakervx-fr.com [/url] Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# thanks to: [url=http://www.66rpg.com]http://www.66rpg.com[/url] for documentation on wininet
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# ! do not use ftp which contains privates data
# ! this scripts need ftp account information !
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# Ftp :
#   â–¼ receive :
# Â Â Â Â Â Net::Ftp.download("dir_on_ftp/file.zip","./Graphics")
#   â–¼ send :
#      Net::Ftp.upload("./Graphics/file.zip","§dir_on_ftp/file.zip")
#   â–¼ make directory :
# Â Â Â Â Â Net::Ftp.mkdir("/dir_on_ftp")
# Http :
#   â–¼ receive :
# Â Â Â Â Â Net::Http.download("url","./Graphics")
#   â–¼ total octets downloaded :
# Â Â Â Â Â Net::HTTP.dloaded
#   â–¼ size of file :
# Â Â Â Â Â Net::HTTP.size("test.zip")
#   â–¼ % dl progress :
# Â Â Â Â Â Net::HTTP.progress("test.zip")
#   â–¼ transfer time:
# Â Â Â Â Â Net::HTTP.temps("test.zip")
#   â–¼ list files :
# Â Â Â Â Â Net::HTTP.transfers
#   â–¼ file loaded? :
# Â Â Â Â Â Net::HTTP.loaded?("test.zip")
#   â–¼ octets transfered :
# Â Â Â Â Â Net::HTTP.transfered
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
module Berka
 module NetError
  ErrConIn="Unable to connect to Internet"
  ErrConFtp="Unable to connect to Ftp"
  ErrConHttp="Unable to connect to the Server"
  ErrNoFFtpIn="The file to be download doesn't exist"
  ErrNoFFtpEx="The file to be upload doesn't exist"
  ErrTranHttp="Http Download is failed"
  ErrDownFtp="Ftp Download is failed"
  ErrUpFtp="Ftp Upload is failed"
  ErrNoFile="No file to be download"
  ErrMkdir="Unable to create a new directory"
 end
end
module Net
 W='wininet'
 SPC=Win32API.new('kernel32','SetPriorityClass','pi','i').call(-1,128)
 IOA=Win32API.new(W,'InternetOpenA','plppl','l').call('',0,'','',0)
 IC=Win32API.new(W,'InternetConnectA','lplpplll','l')
 print(Berka::NetErrorErr::ConIn)if IOA==0
 module FTP
  FSCD=Win32API.new(W,'FtpSetCurrentDirectoryA','lp','l')
  FGF=Win32API.new(W,'FtpGetFileA','lppllll','l')
  FPF=Win32API.new(W,'FtpPutFile','lppll','l')
  FCD=Win32API.new(W,'FtpCreateDirectoryA','lp','l')
  module_function  Â
  def init
   #-=-=-=-=-=-=-=-=-=-=-=-=-=-=-#
   ftp="ftp.server.com"      #
   port=21            #  A modifier !
   identifiant="user"       #
   motdepasse="password"     #
   #-=-=-=-=-=-=-=-=-=-=-=-=-=-=-#
   @fb=IC.call(IOA,ftp,port,identifiant,motdepasse,1,0,0)
   ftp,port,identifiant,motdepasse=[nil]*4 # efface les ids par sécurité
   (print(Berka::NetError::ErrConFtp))if @fb==0
  end
  def download(ext,int='./')
   init if @fb.nil?  Â
   c=ext.split('/').pop
   if FSCD.call(@fb,ext.gsub(c,''))!=0
    print(Berka::NetErrorErr::ErrDownFtp)if FGF.call(@fb,c,"#{int}/#{c}",0,32,2,0)==0
   else
    print(Berka::NetErrorErr::ErrNoFFtpIn)
   end
  end
  def mkdir(rep)
   init if @fb.nil?
   print(Berka::NetErrorErr::ErrMkdir)if FCD.call(@fb,rep)==0
  end
  def upload(int,ext)
   init if @fb.nil?
   if FSCD.call(@fb,ext)&&File.exist?(int)
    print(Berka::NetErrorErr::ErrUpFtp)if FPF.call(@fb,int,ext,2,0)==0
   else
    print(Berka::NetErrorErr::ErrNoFFtpEx)
   end
  end
 end
 #-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
 module HTTP
  IOU=Win32API.new(W,'InternetOpenUrl','lppllp','l')
  IRF=Win32API.new(W,'InternetReadFile','lpip','l')
  ICH=Win32API.new(W,'InternetCloseHandle','l','l')
  HQI=Win32API.new(W,'HttpQueryInfo','llppp','i')
  module_function
  def sizeloaded(i='');@read[i];end
  def transfered;@dloaded;end
  def transfers;@dls;end
  def progress(i='');(@read[i].to_f/@size[i]*100);end
  def loaded?(i='');@read[i]>=@size[i]rescue nil;end
  def temps(i='');@tps[i]if loaded?(i);end
  def size(i='');@size[i];end
  def download(url,int='./')
   @dloaded||=0;@dls||={};@i||=-1;@size||={};@read||={};@tps={}
   a=url.split('/');serv,root,fich=a[2],a[3..a.size].join('/'),a[-1]
   print(Berka::NetErrorErr::ErrNoFile)if fich.nil?
   @dls[fich]=Thread.start(url,int){|url,int|txt='';t=Time.now
   Berka::NetErrorErr::ErrConHttp if(e=IC.call(IOA,serv,80,'','',3,1,0))==0 Â
   f=IOU.call(IOA,url,nil,0,0,0)
   HQI.call(f,5,k="\0"*1024,[k.size-1].pack('l'),nil)
   @read[fich],@size[fich]=0,k.delete!("\0").to_i
   loop do
    buf,n=' '*1024,0
    r=IRF.call(f,buf,1024,o=[n].pack('i!'))
    n=o.unpack('i!')[0]
    break if r&&n==0
    txt << buf[0,n]
    @read[fich]=txt.size
   end
   (File.open(int+fich,'wb')<<txt).close
   @dloaded+=@read[fich]
   ICH.call(f);sleep(0.01)
   @tps[fich]=Time.now-t}
  end
 end
end
Code:
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
#           Téléchargement de fichiers en Rgss
#  par berka            v 2.1           rgss 1
#              www.rpgmakervx-fr.com                     Â
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
# Â Â Â Â Â Â EXEMPLES !
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
class Window_Barre<Window_Base
 def initialize
  super(100,50,440,380)
  self.contents=Bitmap.new(width-32,height-32)
  self.contents.font.name=$fontface
  self.contents.font.size=$fontsize
  # on initialise un tableau de téléchargement
  @dls=Net::HTTP.transfers
  refresh
 end
 def refresh
  i=0
  self.contents.clear
  self.contents.font.size=22
  self.contents.draw_text(-16,0,self.width,24,"Http: Gestionnaire de téléchargement",1)
  self.contents.font.size=20
  # pour chaque téléchargement
  @dls.each_key{|file|
  self.contents.draw_text(0,56*(i+=1),200,24,"--> #{file}")
  self.contents.fill_rect(131,56*i+25,202,10,Color.new(0,0,0,150))
  self.contents.font.size=14
  # on récupere le pourcentage du téléchargement
  pr=Net::HTTP.progress(file)/100
  # on écrit le rapport: octets téléchargés / taille totale
  rat="#{Net::HTTP.sizeloaded(file)/1024}/#{Net::HTTP.size(file)/1024} ko"
  self.contents.draw_text(132,56*i+32,150,24,rat,1)
  # on récupère le temps de téléchargement du fichier
  s=Net::HTTP.temps(file)
  # on l'écrit
  self.contents.draw_text(20,56*i+20,self.width,24,"Fait en #{s} secs")
  # on écrit le pourcentage de progression
  self.contents.draw_text(342,56*i+26,self.width,24,"#{(pr*100).to_i}%")rescue nil
  # et on trace la barre
  self.contents.fill_rect(132,56*i+26,pr*200,8,Color.new(0,255,0))
  self.contents.font.size=20}
 end
end
#-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
class Scene_Download
 def main
  @fond=Spriteset_Map.new
  # chargement des fichiers
  Net::HTTP.download('http://download59.mediafire.com/313lzxpdytpg/gyok0vgmm2m/hero+creator.zip')
  Net::HTTP.download('http://www.google.fr/intl/fr_fr/images/logo.gif')
  Net::HTTP.download('http://www.rpgrevolution.com/forums/banner3.gif')
  Net::HTTP.download('http://i42.servimg.com/u/f42/12/32/03/86/bannie11.jpg')
  #=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-
  @barre=Window_Barre.new
  Graphics.transition
  loop do
   Input.update
   Graphics.update
   update
   break if $scene != self
  end
  Graphics.freeze
  @fond.dispose
  @barre.dispose
 end
 def update
  @barre.refresh
  $scene=Scene_Map.new if Input.trigger?(Input::B)
 end
end
Â
Instructions
The downloaded file has the same name of the originalFtp :
receive : Net::FTP.download("file_on_ftp","./Graphics")
send : Net::FTP.upload("file_in_project","dir_on_ftp")
Http :
receive : Net::HTTP.download("url","./Graphics")
FAQ
Paste this script above main
Compatibility
No Problem I think !
Credits and Thanks
Msdn, my best friend ^^
http://www.66rpg.com for parts of scripts !
Author's Notes
next feature:
evaluate the size of the file: done.
evaluate if the file exists: done.
Terms and Conditions
Free for using, but I need credits. Do not post this script anywhere without my permission !
enjoy,
berka