Chad Sexington
Member
Hello,
I don't know if this is the correct forum. I'm not asking for a full script. I just need help with a few edits.
I know next to nothing of Ruby and I want to replace the EXP information with a line of text and a few pictures. Here's a pair of before & after mock pics of what I'm looking for:
(The EXP information isn't important for my game.)
-I'm using RMXP.
-I'm not using any script that alters the default Status screen.
-The favorite foods for each character never changes through the entire game. So the pictures I want to display are just static and never change.
-Each character has a different set of favorite foods.
-Each character has 2-7 favorite foods.
Can someone provide me with step-by-step instructions on how to do this?
(Is it as easy as I think it is, or is it more involved?)
Will it be easier if I made a single pic for all food items? Or can a series of pics (for each food item) be easier? If it doesn't matter, I'd rather keep them as separate pics and grab them directly from the Graphics/Icons folder.
I'm guessing there will be separate lines I need to add for each character, based on Character IDs. If you provide the code for Character 001 and what and how to add pics, I should be able to figure out the rest.
Any help is appreciated.
I don't know if this is the correct forum. I'm not asking for a full script. I just need help with a few edits.
I know next to nothing of Ruby and I want to replace the EXP information with a line of text and a few pictures. Here's a pair of before & after mock pics of what I'm looking for:
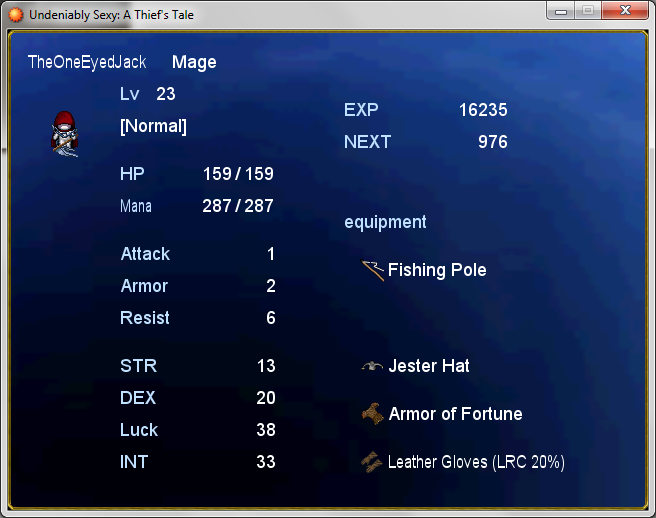
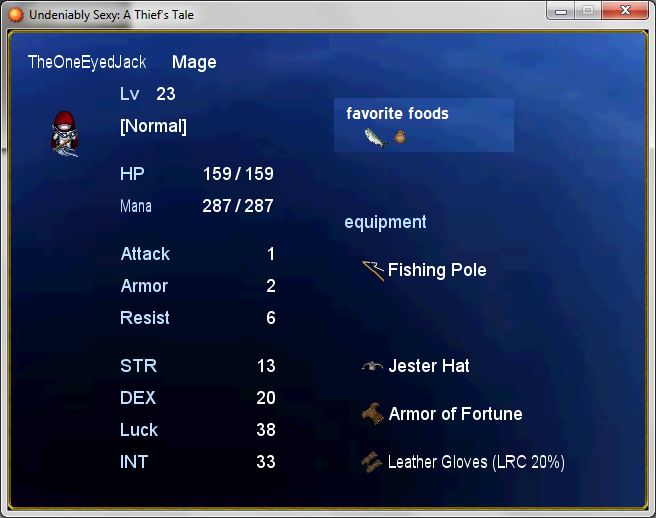
(The EXP information isn't important for my game.)
-I'm using RMXP.
-I'm not using any script that alters the default Status screen.
-The favorite foods for each character never changes through the entire game. So the pictures I want to display are just static and never change.
-Each character has a different set of favorite foods.
-Each character has 2-7 favorite foods.
Can someone provide me with step-by-step instructions on how to do this?
(Is it as easy as I think it is, or is it more involved?)
Will it be easier if I made a single pic for all food items? Or can a series of pics (for each food item) be easier? If it doesn't matter, I'd rather keep them as separate pics and grab them directly from the Graphics/Icons folder.
I'm guessing there will be separate lines I need to add for each character, based on Character IDs. If you provide the code for Character 001 and what and how to add pics, I should be able to figure out the rest.
Any help is appreciated.