Pokémaniac
Awesome Bro
Purpose:
This script allows the map to be scrolled to a certain x, y position. It also allows the map to be scrolled to the player's position.
Instructions:
Paste anywhere above Main to use. No set-up required.
Call $game_map.scroll_to(x posiition, y position, speed) in a call script to scroll to position x, y.
Call $game_map.find_player(speed) in a call script to scroll to the player's position.
Call $game_map.clear_scroll to stop any scroll_to or find_player actions. Use $game_map.clear_scroll(false) to stop any current scrolling as well.
This script is free-to-use. Credits are appreciated but not necessary at all.
To wait for the completion of the scroll, use the following loop:
This loop can be called from a common event and in any type of event except for a parallel process will also stop the player from moving.
Code:
This script allows the map to be scrolled to a certain x, y position. It also allows the map to be scrolled to the player's position.
Instructions:
Paste anywhere above Main to use. No set-up required.
Call $game_map.scroll_to(x posiition, y position, speed) in a call script to scroll to position x, y.
Call $game_map.find_player(speed) in a call script to scroll to the player's position.
Call $game_map.clear_scroll to stop any scroll_to or find_player actions. Use $game_map.clear_scroll(false) to stop any current scrolling as well.
This script is free-to-use. Credits are appreciated but not necessary at all.
To wait for the completion of the scroll, use the following loop:
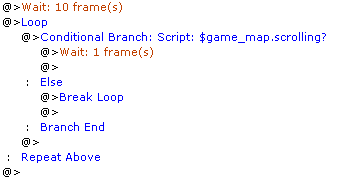
This loop can be called from a common event and in any type of event except for a parallel process will also stop the player from moving.
Code:
Ruby:
###############################################################################
#SCROLL-TO
#by Pokemaniac
#
#Call $game_map.scroll_to(x posiition, y position, speed) in a call script to
#scroll to position x, y.
#
#Call $game_map.find_player(speed) in a call script to scroll to the player's
#position.
#
#Call $game_map.clear_scroll to stop any scroll_to or find_player actions. Use
#$game_map.clear_scroll(false) to stop any current scrolling as well.
#
#This script is free-to-use. Credits are appreciated but not necessary at all.
###############################################################################
class Game_Map
#Initialize variable
alias initialize_scroll_to initialize unless $@
def initialize
initialize_scroll_to
@scrollWaiting = []
end
#Scroll to
def scroll_to(x, y, s = 4)
@scrollWaiting << [x, s, true]
@scrollWaiting << [y, s, false]
end
#Get scroll details
def scroll_details(dis, s, hor)
#if x-axis
if hor == true
dis -= 8
dis -= @display_x / 256
#Get direction
if dis > 0
dir = 6
else
dir = 4
dis *= -1
end
#if y-axis
else
dis -= 6
dis -= @display_y / 256
#Get direction
if dis > 0
dir = 2
else
dir = 8
dis *= -1
end
end
dis = 0 if dis < 0
return [dir, dis, s]
end
#Clear Scroll List
def clear_scroll(finish = true)
@scrollWaiting = []
if !finish
@scroll_rest = 0
end
end
#Return to player
def find_player(speed = 4)
@scrollWaiting << [-1, speed, true]
@scrollWaiting << [-1, speed, false]
end
#Change refresh
alias update_scroll_to update unless $@
def update
update_scroll_to
#If not already scrolling and if there are more scroll commands
if !scrolling?
if @scrollWaiting == nil
@scrollWaiting = []
elsif !@scrollWaiting.empty?
newScroll = @scrollWaiting[0]
#If scroll to player
if newScroll[0] == -1
if newScroll[2] == true
xy = $game_player.x
else
xy = $game_player.y
end
else
xy = newScroll[0]
end
newScroll = scroll_details(xy, newScroll[1], newScroll[2])
dir = newScroll[0]
dis = newScroll[1]
spe = newScroll[2]
start_scroll(dir, dis, spe)
@scrollWaiting.shift
end
end
end
end